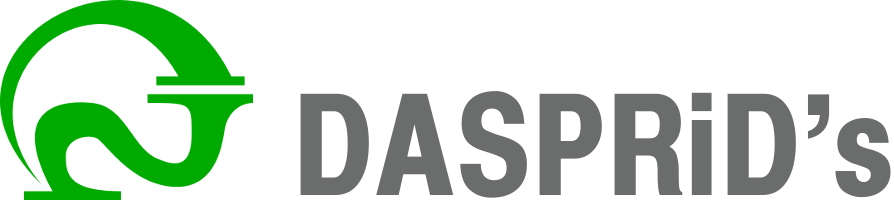
Ben Scholzen
Архітэктар праграмнага забеспячэння і кансультант
Ben Scholzen is …
de-CH.utf-8 (old style: de_CH.utf-8)
nplurals=5; plural=n==1 ? 0 : n==2 ? 1 : n < 7 ? 2 : n < 11 ? 3 : 4
return array( '' => array( 'plural_forms' => 'nplurals=2; plural=n!=1' ), 'bacon' => 'speck', '%d house' => array( 'Ein Haus', '%d Häuser' ) );
use Zend\I18n\Translator\Translator; $translator = new Translator::factory(array( 'locale' => 'de_DE', 'files' => array( array( 'type' => 'phparray', 'filename' => 'languages/de-DE.php' ) ) ));
Simple string:
echo $translator->translate('bacon'); // speck
String with plural form:
$houses = 5; printf( $translator->translatePlural('%d house', '%d houses', $houses), $houses ); // 5 Häuser
<?php echo $this->translate('bacon'); ?>
<?php printf( $this->translatePlural('%d house', '%d houses', $number), $number ); ?>
Switch text domain for a single call
<?php echo $this->translate('bacon', 'module-b'); ?>
Switch text domain for all following calls
<?php $this->plugin('translate')->setTranslatorTextDomain('module-b'); ?>
<?php echo $this->translate('bacon', null, 'fr-FR'); ?>
use Zend\I18n\Translator\Translator; $translator = new Translator::factory(array( 'locale' => 'de_DE', 'patterns' => array( array( 'type' => 'phparray', 'base_dir' => 'translations', 'pattern' => '%s.php' ) ) ));
$view->plugin('dateFormat')->setLocale('en-US');
$view->plugin('dateFormat')->setTimezone('Europe/Berlin');
<?php echo $this->dateFormat( $date, $dateType, // Optional, default: NONE $timeType, // Optional, default: NONE $locale // Optional ); ?>
$formatter = new \IntlDateFormatter( $locale, $dateType, $timeType /*, $timezone*/ ); $formattedDate = $formatter->format($date);
$formatter = new \IntlDateFormatter(/* … */); $timestamp = $formatter->parse($formattedDate); $date = new \DateTime('@' . $timestamp);
$view->plugin('numberFormat')->setLocale('en-US'); $view->plugin('currencyFormat')->setLocale('en-US');
<?php echo $this->numberFormat( $number, $formatStyle, // Optional, default: DECIMAL $formatType, // Optional, default: TYPE_DEFAULT $locale // Optional ); ?>
<?php echo $this->currencyFormat( $number, $currencyCode, // Optional, default: not set $showDecimals, // Optional, default: true $locale // Optional ); ?>
$view->plugin('numberFormat')->setFormatStyle($formatStyle); $view->plugin('numberFormat')->setFormatType($formatType);
$view->plugin('currencyFormat')->setCurrencyCode($currencyCode); $view->plugin('currencyFormat')->setShouldShowDecimals($boolean);
$formatter = new \NumberFormatter( $locale, $style ); $formattedNumber = $formatter->format($number);
$formatter = new \NumberFormatter(/* … */); $number = $formatter->parse($formattedNumber);
$filter = new \Zend\I18n\Filter\NumberFormat($locale, $type, $style); $number = $filter->filter($formattedNumber);
Rate this talk on joind.in: https://joind.in/7020