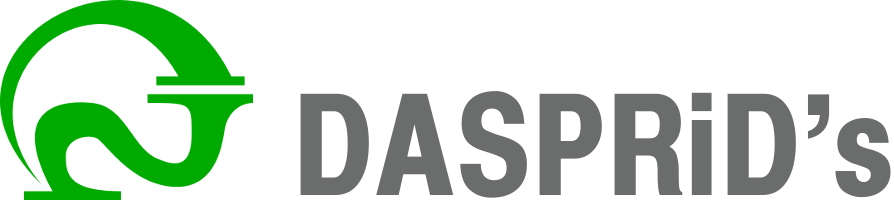
Ben Scholzen is …
namespace Zend\Mvc\Router; use Zend\Stdlib\RequestInterface as Request; interface RouteInterface { public static function factory($options = array()); public function match(Request $request); public function assemble( array $params = array(), array $options = array() ); }
use Zend\Mvc\Router\Http\TreeRouteStack; $router = TreeRouteStack::factory(array( 'routes' => array( // … ) ));
or
$router = new TreeRouteStack(); $router->addRoutes(array(/* … */));
$router->setBaseUrl('/my/application');
use Zend\Uri\Http as HttpUri; $router->setRequestUri(new HttpUri('http://example.com'));
$router->setDefaultParam('key', 'value);
$router->setDefaultParams(array( 'key' => 'value' ));
$url = $router->assemble( array(), // Params array('name' => 'bacon') // Options ); echo $url; // /bacon
$url = $router->assemble( array(), // Params array( 'name' => 'bacon', 'force_canonical' => true, ) ); echo $url; // http://example.com/bacon
use Zend\Http\PhpEnvironment\Request; $request = new Request(); $routeMatch = $router->match($request); $routeMatch->getMatchedRouteName(); $routeMatch->getParams(); $routeMatch->getParam($name, $default = null);
array( 'articles' => array( 'type' => 'literal', 'options' => array( 'route' => '/articles', 'defaults' => array( 'controller' => 'Application\Controller\Article', 'action' => 'index', ) ) ) )
array( 'article-details' => array( 'type' => 'regex', 'options' => array( 'route' => '/articles/(?P<id>\d+)', 'spec' => '/articles/%id%', 'defaults' => array( 'controller' => 'Application\Controller\Article', 'action' => 'details', ) ) ) )
array( 'wildcard' => array( 'type' => 'wildcard', 'options' => array( 'key_value_delimiter' => '/', 'param_delimiter' => '/' ) ) )
array( 'article-details' => array( 'type' => 'segment', 'options' => array( 'route' => '/articles[/:id]', 'constraints' => array('articles' => '\d+'), 'defaults' => array( 'controller' => 'Application\Controller\Article', 'action' => 'index', ) ) ) )
/controller/action/key1/value1/key2/value2 …
namespace Zend\Mvc\Router\Http; use Zend\Mvc\Router\RouteInterface as BaseRoute; interface RouteInterface extends BaseRoute { public function getAssembledParams(); }
array( 'articles' => array( 'type' => 'literal', 'options' => array( 'route' => '/articles', 'defaults' => array( 'controller' => 'Application\Controller\Article', 'action' => 'index' ) ), 'may_terminate' => true, 'child_routes' => array( 'details' => array( 'type' => 'segment', 'route' => '/:id', 'constraints' => array('articles' => '\d+'), 'defaults' => array('action' => 'details') ) ) ) )
$router->assemble( array(), array('name' => 'articles/details') );
array( 'user' => array( 'type' => 'hostname', 'options' => array( 'route' => ':user.users.example.com', 'defaults' => array( 'controller' => 'Application\Controller\User', 'action' => 'index', ) ) ) )
array( 'secure' => array( 'type' => 'scheme', 'options' => array( 'scheme' => 'https' ) ) )
array( 'non-get' => array( 'type' => 'method', 'options' => array( 'verb' => 'post,put' ) ) )
array( 'query' => array( 'type' => 'query' ) )
return array( 'router' => array( 'routes' => array( // … ) ) );
class BaconController extends AbstractActionController { public function indexAction() { $id = $this->params('id'/*, null */); } }
class BaconController extends AbstractActionController { public function indexAction() { $this->redirect()->toRoute( 'route-name', array(), // Params array() // Options ); } }
class BaconController extends AbstractActionController { public function indexAction() { $url = $this->url( 'route-name', array(), // Params array() // Options ); $this->redirect()->toUrl($url . '#hash-tag'); } }
<a href="<?php echo $this->url( 'route-name', array(), // Params array() // Options ); ?>">Some Link</a>
Rate this talk on joind.in: https://joind.in/6872